Published
- 2 min read
How to Dynamically Populate Tailwind CSS Property Values
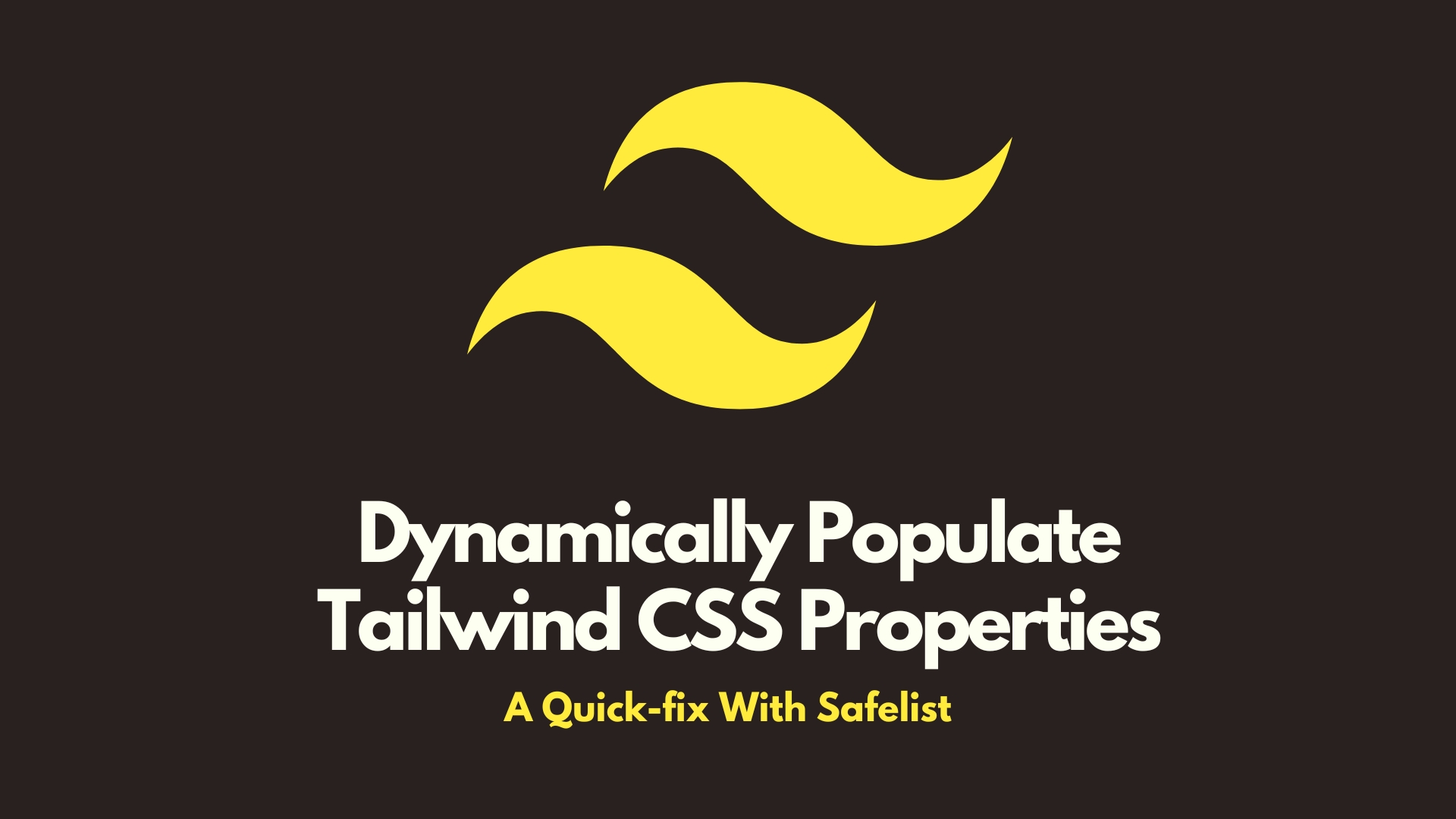
Tailwind CSS is an excellent utility-first CSS framework that makes styling web applications a breeze. However, one challenge I’ve faced is dynamically applying styles based on conditions or props, especially when dealing with Tailwind’s class optimizations. In this blog post, I’ll delve into how to dynamically apply styles in Tailwind CSS and ensure they are preserved during the build process by using the safelist feature.
The Problem: Dynamic Style Application in Tailwind CSS
Consider a scenario where I want to create a flexible grid layout in a React component, adjusting the number of columns dynamically based on the number of children elements. Here’s an example:
const Row = ({ children }) => {
return <div className={`grid grid-cols-${React.Children.count(children)}`}>{children}</div>
}
In this code snippet, I attempt to dynamically set the grid-cols
class based on the number of child elements. However, this approach does not work as expected with Tailwind CSS.
Why It Doesn’t Work
Tailwind CSS optimizes and purges unused styles during the build process. It analyzes the code for class names and eliminates any that are not statically defined. Since grid-cols-${React.Children.count(children)}
is not a static class name, Tailwind doesn’t recognize it and discards it during optimization.
The Solution: Using Safelist in Tailwind Config
To solve this issue, I needed to ensure Tailwind CSS is aware of the dynamic class names I intend to use. This is where the safelist feature comes into play. By adding a pattern to the safelist, I can instruct Tailwind to preserve these dynamically generated class names.
Step-by-Step Implementation
-
Identify the Pattern: Determine the pattern of the class names I need to safelist. In this case, it’s
grid-cols-
followed by a number. -
Update Tailwind Configuration: Modify the
tailwind.config.js
(ortailwind.config.ts
if you’re using TypeScript) to include this pattern in the safelist.
Here’s how I did it:
// tailwind.config.js
module.exports = {
// other configurations...
safelist: [
{
pattern: /grid-cols-(\d+)/
}
]
}
Understanding the Regex Pattern
The regex /grid-cols-(\d+)/
matches any class name that starts with grid-cols-
followed by one or more digits. This ensures that Tailwind preserves any grid column classes I dynamically generate, such as grid-cols-1
, grid-cols-2
, and so on.
Conclusion
Dynamic styling in Tailwind CSS can be challenging due to its aggressive optimization and purging strategies. However, by leveraging the safelist feature, I can instruct Tailwind to retain specific patterns of class names, ensuring my dynamic styles are applied correctly. This approach not only solves the problem but also maintains the efficiency and performance benefits of using Tailwind CSS.