Published
- 3 min read
How to Extend the Express Request Object Type with TypeScript
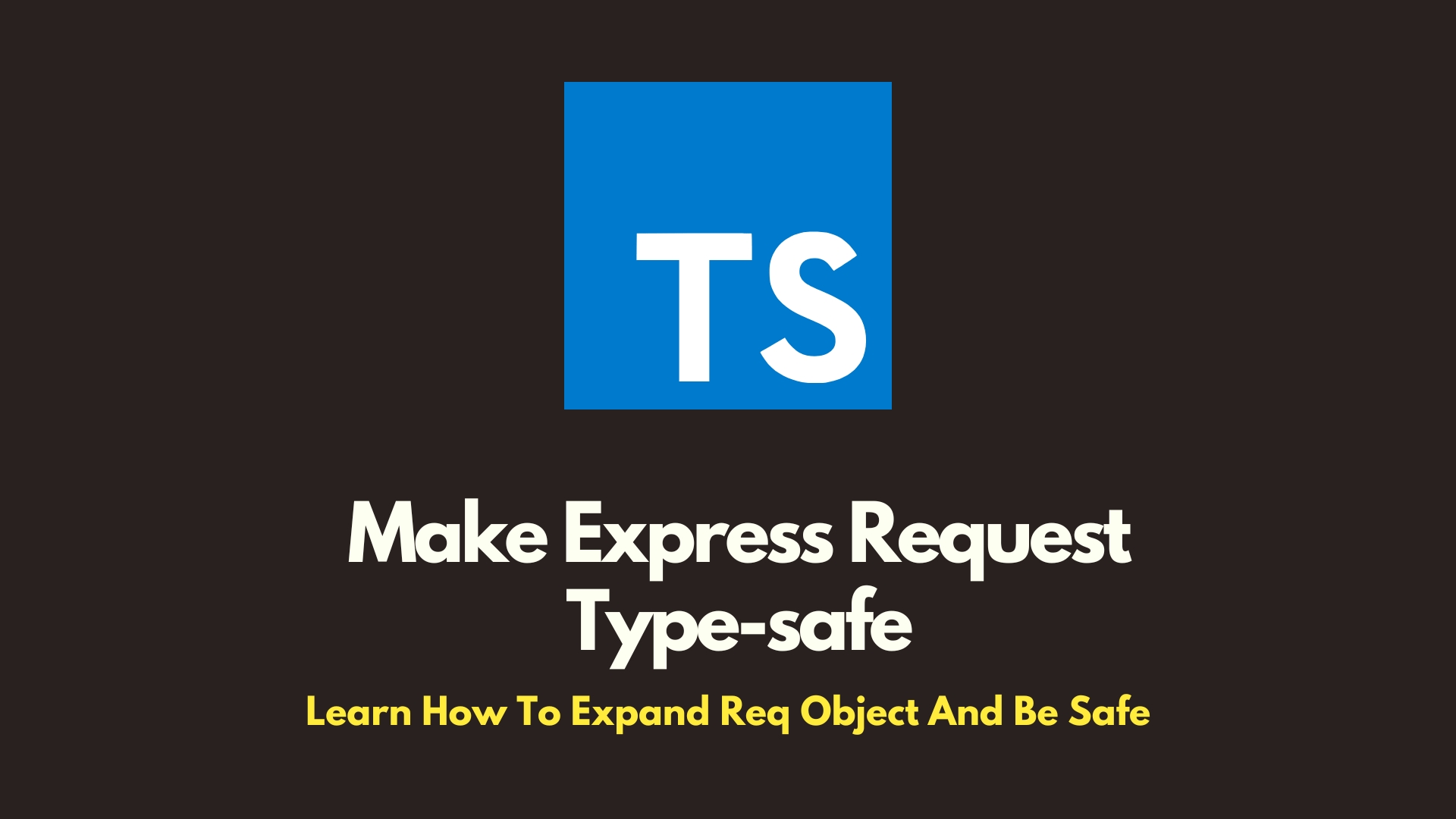
In modern web development, TypeScript has become a staple for developers who want to add static type-checking to their JavaScript code. This additional layer of type safety can help catch errors early in the development process and make code more maintainable. When working with Express.js, one common scenario developers encounter is the need to extend the request object to include additional properties, such as user information added by middleware. In this blog post, we’ll explore how to extend the request object’s type using TypeScript.
Understanding the Request Object
In an Express.js application, the request object is a central piece of middleware functions. It provides access to the HTTP request and contains information such as the request method, URL, headers, and more. However, there are times when you need to augment this object with additional properties. For instance, after authenticating a user, you might want to attach the user’s ID to the request object for further processing in subsequent middleware or route handlers.
By default, TypeScript doesn’t know about these additional properties, which can lead to errors when trying to access them. To solve this, we need to extend the type definition of the request object.
Extending the Request Object’s Type
To extend the request object, we need to modify the global Express
namespace. Here’s how we can achieve this:
-
Create a Custom Type Definitions Directory
First, create a new directory at the root of your project to hold custom type definitions. A common convention is to name this directory
@types
.mkdir @types
-
Extend the Express Package
Within the
@types
directory, create a subdirectory namedexpress
. This will allow us to extend the express package’s types.mkdir @types/express
-
Create an Index File for Type Definitions
Inside the
express
directory, create a file namedindex.d.ts
. This file will contain the type extensions for the Express request object.// @types/express/index.d.ts import * as express from 'express' declare global { namespace Express { interface Request { userId?: string isSubscriber?: boolean ...any other field you might attach to request object } } }
In this code snippet, we’re extending the
Request
interface to include two optional properties:userId
andisSubscriber
. Thedeclare global
syntax is used to add these properties to the globalExpress
namespace, making them available throughout your application. -
Configure TypeScript to Use Custom Type Definitions
Finally, update your
tsconfig.json
file to include the@types
directory in thetypeRoots
array. This tells TypeScript to look in this directory for additional type definitions.{ "compilerOptions": { // ...other options... "typeRoots": ["@types"] } }
By default, TypeScript only looks in
node_modules/@types
for type definitions. Adding our custom@types
directory ensures that TypeScript recognizes the additional type definitions we’ve created.
Why Extend the Request Object?
Extending the request object offers several benefits:
-
Type Safety: By extending the request object’s type, TypeScript will know about the additional properties and can provide compile-time checks. This reduces the risk of runtime errors caused by accessing undefined properties.
-
Improved Developer Experience: With type definitions in place, editors can offer better IntelliSense, making it easier for developers to discover and use the additional properties.
-
Maintainability: Clearly defining the shape of the request object helps maintain consistency across the application, especially in large codebases where multiple developers are working together.
Conclusion
Extending the request object’s type in an Express application using TypeScript is a straightforward process that enhances the robustness and maintainability of your code. By following the steps outlined in this post, you can easily add custom properties to the request object and take full advantage of TypeScript’s type-checking capabilities. As you continue to develop your application, these practices will help you catch errors early and improve the overall quality of your code.